Overview
TuitionConnect is a desktop address book application used by private tuition teacher. It aims to help the tutors to manage their busy schedule more efficiently and effectively. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 20 kLoC.
Summary of contributions
-
Major enhancement: integrated CalendarFX into the application
-
What it does: has a calendar that reflects all the task entries and respond to all scheduling commands that the user inputs.
-
Credits: [CalendarFX]
-
-
Minor enhancement:
-
Constructed classes such as PersonalTask, TuitionTask, Tutee, Subject, Grade, EducationLevel, School and relevant methods to support task and tutee management commands.
-
Modified edit command to be able to edit tutee’s details.
-
Added addtutee command to add a new tutee into TuitionConnect application. #62
-
Drafted a TuitionConnect UI theme and revamped the layout of the UI.
-
-
Code contributed: [Functional code] [Test code]
-
Other contributions:
-
Drafted and managed user guide in developer guide and on GitHub: #106
-
Responsible for issues and milestones on GitHub
-
Set up auto publishing of documentation on GitHub
-
Reported several bugs for other teams in class: (Example: [Endless Loop]https://github.com/CS2103JAN2018-W10-B3/main/issues/112)
-
Introduction
TuitionConnect (TC) is an integration of a personalised address book, task manager and income tracker for private home tutors.
Stems from our strong passion in simplifying the life of tutors, TuitionConnect introduces features
to aid management of tutee contacts, tuition schedule and income tracking. Moreover, we believe that a thorough connectivity is the
root of simplicity. Hence, there are also features which support the management of your personal contacts and tasks.
TuitionConnect is primarily for tutors who prefer to use a desktop app for managing contacts and tasks.
More importantly, TuitionConnect is optimized for those who prefer to work with a Command Line Interface
(CLI) while still having the benefits of a Graphical User Interface (GUI).
With TuitionConnect’s keyboard shortcuts and productive features, TuitionConnect can get your contact and task management done faster than traditional GUI apps. Interested? Jump to the [Quick Start] to get started. Enjoy!
Adding a tutee: addtutee
Adds a tutee to the address book
Using Command Word:
Format: addtutee n/NAME p/PHONE_NUMBER e/EMAIL a/ADDRESS s/SUBJECT g/GRADE edu/EDUCATION_LEVEL sch/SCHOOL [t/TAG]…
|
|
Examples:
-
addtutee n/John Doe p/98765432 e/johnd@example.com a/John street, block 123, #01-01 s/Economics g/B+ edu/junior college sch/Victoria Junior College
-
addtutee n/Betsy Crowe s/Mathematics g/C e/betsycrowe@example.com a/Newgate Town p/1234567 t/owesMoney sch/Victoria Institution edu/secondary
-
addtutee n/Dickson ee p/91234567 e/dickson@exmaple.com a/Dickson street, block 456, #02-02 s/english g/f9 edu/primary sch/Newgate Primary School
Editing a contact : edit
Edits existing contact’s details in the address book.
Using Command Word:
Format: edit INDEX [n/NAME] [p/PHONE] [e/EMAIL] [a/ADDRESS] [s/SUBJECT] [s/SUBJECT] [g/GRADE] [edu/EDUCATION_LEVEL] [sch/SCHOOL] [t/TAG]…
Using Comamnd Alias:
Format: e INDEX [n/NAME] [p/PHONE] [e/EMAIL] [a/ADDRESS] [s/SUBJECT] [s/SUBJECT] [g/GRADE] [edu/EDUCATION_LEVEL] [sch/SCHOOL] [t/TAG]…
Keyword | Description |
---|---|
edit |
To call the edit command |
INDEX |
Index number shown in the last person listing. It must be a positive integer 1, 2, 3, … |
INDEX |
The contact at the specified |
|
Valid Details to edit for each type of contact:
Person | Tutee |
---|---|
NAME |
NAME |
PHONE |
PHONE |
ADDRESS |
ADDRESS |
--- |
SUBJECT |
--- |
GRADE |
--- |
EDUCATION_LEVEL |
--- |
SCHOOL |
TAG |
TAG |
|
Examples:
-
edit 1 p/91234567 e/johndoe@example.com
Edits the phone number and email address of the 1st contact to be91234567
andjohndoe@example.com
respectively. -
e 2 n/Betsy Crower sch/JohnDoe Secondary t/
Edits the name of the 2nd contact (a tutee) to beBetsy Crower
, her school toJohnDoe Secondary
and clears all existing tags (except "Tutee" tag).
Changing calendar view page time unit: change
Changes the calendar’s view page into the time unit specified by you.
Using Command Word:
Format: change TIME_UNIT
Time Unit | User input | Description |
---|---|---|
Day |
d |
View the calendar in day |
Week |
w |
View the calendar in week |
Month |
m |
View the calendar in month |
Year |
y |
View the calendar in year |
|
Examples:
-
change d
(command fails as current calendar view is already in day) -
change w
(changes calendar view to week) -
change m
(changes calendar view to month) -
change y
(changes calendar view to year)Year view is unable to display all the months due to third party software constraint.
Update tutee fees [coming in v2.0]
Updates the remaining balance of the tuition fees of a specific tutee after he or she has paid.
Complete task [coming in v2.0]
Marks a task as completed. If the task is tuition, fees will automatically be added to the tutee’s fee balance.
Edit task [coming in v2.0]
Edits date, time, duration or description of an existing task and reflects the changes on the calendar.
View contact address on Google map [coming in v2.0]
Views the location of a specific contact’s address on google map and the shortest route from the current location will be displayed.
Select a contact or a task [coming in v2.0]
Selects either a contact or a task and a pop up page containing all the relevant information will appear.
Contributions to the Developer Guide
Add tutee Command
Current Implementation
Reason for Implementation
As the user of this application is a private tutor, he or she will need to add the tutees' contact details. Since a tutee contains more details specific to them compared to a person, tutee contacts should not be mixed with the person contacts so that all the contacts are organized and can be managed easily. Therefore, AddTuteeCommand is used to add only the tutees' details.
How it is implemented
The AddTuteeCommand is an extension of the Undoable Command, which is part of the Logic Component. As this command involves the altering of the application’s state, the ability to undo and redo will make this command more user-friendly and efficient. (e.g list command + delete command vs undo command).
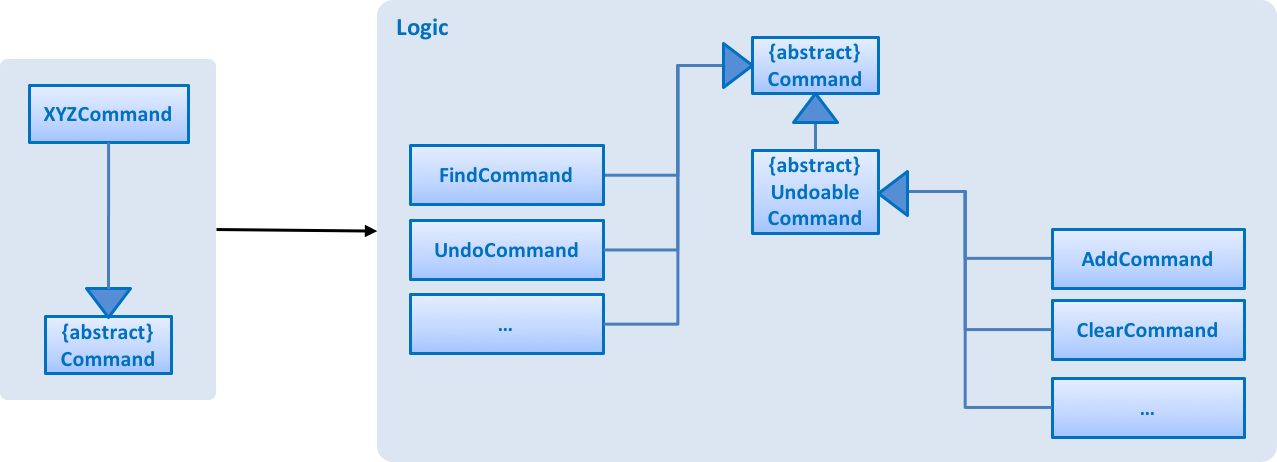
From the diagram shown above, instead of extending abstract Command class directly, AddTuteeCommand will extend the abstract Undoable Command class like clearCommand and AddCommand. This allows AddTuteeCommand to use or override the state saving codes inside the Undoable Command class as shown below.
public abstract class UndoableCommand extends Command {
@Override
public CommandResult execute() {
// ... undo logic ...
executeUndoableCommand();
}
}
public class AddTuteeCommand extends UndoableCommand {
@Override
public CommandResult executeUndoableCommand() {
// ... add tutee logic ...
}
}
The following sequence diagram shows how the addtutee operation works:

As shown in the sequence diagram, after Tutee object has been created, it is being passed to Model. Model manager will add the Tutee object to the person list in TuitionConnect. By having a combined list of tutee and person, we can manage the contacts using one command, such as delete command, instead of having 1 command for tutee and 1 command for person. The combined list is achieved through the use of inheritance, whereby Tutee class inherits the Person class as shown in the diagram below.
Since a tutee is a person, we can carried out inheritance by extending the Person class. Both Tutee and Person class objects have the same fields such as Name, Phone, Email and Address. However, Tutee class object has additional details such as Subject, Grade, EducationLevel and School, which are irrelevant to a Person class object.
If the person already exists, either as tutee or person, in the contact list, DuplicatePersonException will be thrown. |
The following activity diagram shows the workflow when adding a tutee.
Design Consideration
-
Alternative 1 (current choice): Create a new command "addtutee" and a tutee class, which extends person class
-
Pros: Person will not have any detail that is specific to tutee only. This saves memory especially when there are numerous person objects.
-
Cons: A tutee cannot simply convert into a person object when tutor stops teaching him/her. Tutor has to delete the tutee and add him/her again as a person.
-
-
Alternative 2: Modify the current AddCommand and add more fields to the person class
-
Pros: Features can be implemented easily without modifying much to the existing code.
-
Cons: Person object creates unrelated field objects such as "subject" and "school" and these objects will waste the memory space. This problem will be significant when there are a lot of person objects.
-